4.4 Special Files
1. Overview
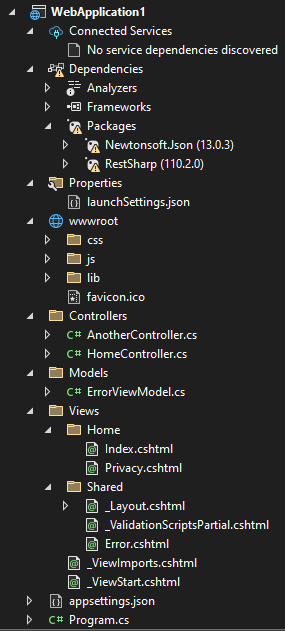
In ASP.NET MVC, the _Layout.cshtml
file is a layout file used to define a common structure (like a master page) for multiple views. It contains the HTML skeleton, including sections like header, footer, and navigation, that can be reused across multiple pages. Child views can inject their own content into the layout using the @RenderBody
or @RenderSection
methods.
Other special files:
- _ViewStart.cshtml: Runs before any view is rendered and typically sets the layout for the views.
- _ViewImports.cshtml: Used to import common namespaces, tag helpers, or directives that should be available in all views.
- _ValidationScriptsPartial.cshtml: Includes client-side validation scripts in your views.
- Web.config (in Views folder): Configuration settings specific to Razor views.
2. Details About The Files
Following sections details the examples that demonstrates the use of special files like _Layout.cshtml
, _ViewStart.cshtml
, and a typical view in ASP.NET MVC.
Example Project Structure:
- …
DirectoryViews
DirectoryHome
- Index.cshtml
DirectoryShared
- _Layout.cshtml
- _ValidationScriptsPartial.cshtml
- _ViewImports.cshtml
- _ViewStart.cshtml
- …
- _Layout.cshtml defines the overall page structure (header, footer, etc.).
- _ViewStart.cshtml ensures all views use the layout file by default.
- _ViewImports.cshtml imports namespaces and helpers globally.
- Index.cshtml is a child view that provides its content and optionally includes custom scripts.
2.1 _Layout.cshtml (Layout File)
This is the layout that defines the structure of your page. It contains the common elements like the navigation bar, footer, etc.
<!DOCTYPE html><html lang="en"><head> <meta charset="utf-8" /> <title>@ViewBag.Title - My MVC Application</title> <link href="~/Content/bootstrap.css" rel="stylesheet" /> <script src="~/Scripts/jquery-3.4.1.min.js"></script></head><body> <header> <nav> <ul> <li><a href="@Url.Action("Index", "Home")">Home</a></li> <li><a href="@Url.Action("About", "Home")">About</a></li> <li><a href="@Url.Action("Contact", "Home")">Contact</a></li> </ul> </nav> </header>
<!-- Main Content --> <div class="container"> @RenderBody() <!-- This will render the content from the child views --> </div>
<footer> <p>© @DateTime.Now.Year - My MVC Application</p> </footer>
<!-- Optional Section Rendering --> @if (IsSectionDefined("Scripts")) { @RenderSection("Scripts", required: false) <!-- For custom scripts in child views --> }</body></html>
@RenderBody()
: This is where the child view content will be injected.@RenderSection("Scripts")
: Optional section where views can provide their custom scripts.
2.2 _ViewStart.cshtml
This file ensures that all views use the layout defined in _Layout.cshtml
by default.
@{ Layout = "~/Views/Shared/_Layout.cshtml"; // Path to the layout file}
- This means that any view that doesn’t specify a different layout will automatically use
_Layout.cshtml
.
2.3 _ViewImports.cshtml
This file is used to import namespaces and helpers that will be available across all views.
@using MyMvcApp.Models@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
@using
: Adds common namespaces so that you don’t have to add them in every view.@addTagHelper
: Enables tag helpers, like form validation, throughout the application.
2.4 Child View (Index.cshtml)
This is a typical view that will use the layout file and provide specific content.
@{ ViewBag.Title = "Home Page";}
<h2>Welcome to the Home Page</h2><p>This is the main content of the home page.</p>
@section Scripts { <script> console.log("Custom script for the Home page"); </script>}
ViewBag.Title
: This is a dynamic property that passes the page title to the layout.@section Scripts
: This injects custom JavaScript code specifically for the Home page and will be rendered where@RenderSection("Scripts")
is in the layout.
2.5 _ValidationScriptsPartial.cshtml
This partial view includes client-side validation scripts. It’s commonly added to forms for validation support.
<script src="~/Scripts/jquery.validate.min.js"></script><script src="~/Scripts/jquery.validate.unobtrusive.min.js"></script>
You can include it in a form view like this:
@Html.Partial("_ValidationScriptsPartial")