4.1 Web MVC
ASP.NET MVC, .NET Package Manager Basics
Creating a ASP.NET MVC project will show the following folder structure
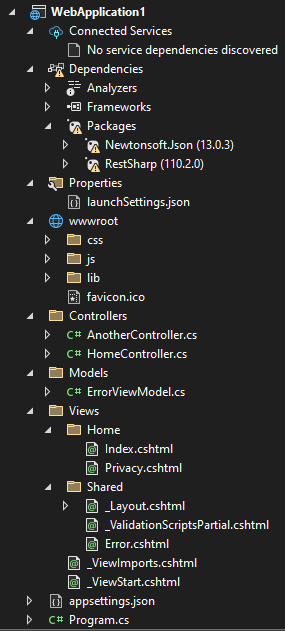
1. Model
-
The Model represents the application’s data and business logic.
-
It is responsible for retrieving and storing data, as well as implementing the business rules and logic that manipulate the data.
-
Models typically consist of classes that define the structure of your data entities, often interacting with databases or external data sources.
-
ASP.NET Core supports various data access technologies, such as Entity Framework Core, to work with databases seamlessly.
- …
DirectoryModels
- ErrorViewModel.cs a model file
DirectoryViews
DirectoryHome
- Index.cshtml
- Privacy.cshtml
- …
Models/ErrorViewModel.cs public class ErrorViewModel{public string? RequestId { get; set; }public bool ShowRequestId => !string.IsNullOrEmpty(RequestId);}-
RequestId{ get; set; }
: This line defines a public property namedRequestId
. The property has a getter and a setter. The type of the property isstring?
, where the?
indicates that the property can be nullable. This property is intended to hold a unique identifier for the user’s request that resulted in an error. This identifier can help with debugging and tracking down specific issues. -
public bool ShowRequestId => !string.IsNullOrEmpty(RequestId);
: This is a computed property (read-only property with a calculated value) namedShowRequestId
. It calculates its value based on whether theRequestId
property is not null or empty. IfRequestId
has a value,ShowRequestId
will be true, indicating that the identifier should be shown to the user. If RequestIdis null or empty,ShowRequestId
will be false
2. View
- The View is responsible for presenting data to users.
- It’s the user interface that users interact with. Views are usually created using markup languages like HTML, along with embedded server-side code to render dynamic content.
- In ASP.NET Core MVC, views are often associated with the Razor view engine, which combines HTML and C# code to create dynamic web pages.
-
The
shared folder
has views which are shared by the individual cshtmlDirectoryModels
- ErrorViewModel.cs
DirectoryViews
DirectoryHome
- Index.cshtml
- Privacy.cshtml
DirectoryShared
- _Layout.cshtml
- _ValidationScriptsPartial.cshtml
- Error.cshtml
- …
Home/Index.cshtml @{// In this block, C# code can be written.// Set the following to display the page title on the browser tab.ViewData["Title"] = "Home Page";}<div class="text-center"><h1 class="display-4">Welcome</h1><p>Learn about <a href="https://docs.microsoft.com/aspnet/core">building Web apps with ASP.NET Core</a>.</p></div>Home/Privacy.cshtml @{ViewData["Title"] = "Privacy Policy";}<h1>@ViewData["Title"]</h1><p>Use this page to detail your site's privacy policy.</p>
3. Controller
-
The Controller acts as an intermediary between the Model and the View.
-
It receives user requests, processes them, interacts with the Model to retrieve data, and then selects the appropriate View to render the response.
-
Controllers contain action methods that handle specific user interactions. These action methods are responsible for coordinating the data flow between the Model and the View
DirectoryControllers
- AnotherController.cs
- HomeController.cs
DirectoryModels
- ErrorViewModel.cs
DirectoryViews
DirectoryHome
- Index.cshtml
- Privacy.cshtml
- …
Controllers/HomeController.cs // Controller class must end with "Controller" suffixpublic class HomeController : Controller{private readonly ILogger<HomeController> _logger;public HomeController(ILogger<HomeController> logger){_logger = logger;}// This method is an action method for the "Home/index.cshtml" page.public IActionResult Index(){// Calling `view()` inside `Index()` method will look for `Home/index.cshtml`// [controller]/index.cshtmlreturn View();}// This method is an action method for the "Home/privacy.cshtml" page.public IActionResult Privacy(){// Calling `view()` inside `Privacy()` method will look for `Home/privacy.cshtml`// [controller]/privacy.cshtmlreturn View();}[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]public IActionResult Error(){return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });}}-
public IActionResult Index()
: This method is an action method for the “Index” page. It returns anIActionResult
, which is the base class for all possible action results. In this case, it returns the result of theView()
method, indicating that the “Index” view should be rendered. -
public IActionResult Privacy()
: Similar to the “Index” method, this action method returns the result of theView()
method, indicating that the “Privacy” view should be rendered.
Controllers/AnotherController.cs // Controller must end with "Controller" suffixpublic class HomeController : Controller{private readonly ILogger<HomeController> _logger;public HomeController(ILogger<HomeController> logger){_logger = logger;}// This method is an action method for the "Home/index.cshtml" page.public IActionResult Index(){// Calling `view()` inside `Index()` method will look for `Home/index.cshtml`// [controller]/index.cshtmlreturn View();}// This method is an action method for the "Home/privacy.cshtml" page.public IActionResult Privacy(){// Calling `view()` inside `Privacy()` method will look for `Home/privacy.cshtml`// [controller]/privacy.cshtmlreturn View();}[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]public IActionResult Error(){return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });}}
4. Routing
4.1 Convention-based Routing
-
Convention-based routing is a routing mechanism in ASP.NET Core MVC that allows you to define routes based on conventions and patterns.
-
Default Route: Convention-based routing often starts with a default route. The default route template specifies placeholders for the controller and action names, along with optional route parameters. This route acts as a catch-all for incoming requests that don’t match any specific routes.
-
Controller and Action Naming: The framework assumes certain naming conventions for controllers and actions. For example, a URL segment like
/Home/Index
is automatically mapped to theIndex()
action within theHomeController
. -
Route Parameters: Convention-based routing also handles route parameters, allowing you to capture values from the URL and pass them to action methods. For example, a URL like
/Products/Details/5
maps to theDetails
action within theProductsController
and passes the value5
as a parameter.
DirectoryModels
- ErrorViewModel.cs
DirectoryViews
DirectoryHome
- Index.cshtml
- Privacy.cshtml
DirectoryShared
- _Layout.cshtml
- _ValidationScriptsPartial.cshtml
- Error.cshtml
- …
- Program.cs
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.// Can wire more services (plugins)// This section is usually used to wire dependancy injectionbuilder.Services.AddControllersWithViews();
var app = builder.Build();
// Configure the HTTP request pipeline.if (!app.Environment.IsDevelopment()){ app.UseExceptionHandler("/Home/Error");}app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
// Configure routing
// Optional: Adding a new MapControllerRoute// app.MapControllerRoute(// name: "api",// pattern: "api/{controller}/{actiopn}/{id?}"//)// Here, a new custom route named "api" is added. This route template defines a pattern that starts with "api/" followed by the controller, action, and an optional id parameter. So, URLs like `/api/students/details/5` would match this route and be mapped to the appropriate controller action.
app.MapControllerRoute( name: "default", pattern: "{controller=Home}/{action=Index}/{id?}"); // Will call action `Index()` method in HomeController // This route acts as a catch-all for incoming requests that don't match any specific routes.
app.Run();
4.2 Attribute Routing
-
Enable Attribute Routing: In ASP.NET Core, Attribute Routing is enabled by default. In earlier versions of ASP.NET MVC, you need to enable it by calling the MapMvcAttributeRoutes method in the RouteConfig or Startup class.
-
Controller-Level Routing: You can apply a route template at the controller level using the [Route] attribute on the controller class. This template will be the prefix for all action methods within the controller.
-
Action-Level Routing: You can further customize the route for individual action methods using the [HttpGet], [HttpPost], or other HTTP method attributes combined with the [Route] attribute.
5. Launch Settings
launchSettings.json
: This file is commonly found in ASP.NET Core projects and is used to configure how the application is launched during development, including various settings for debugging, environment variables, and more.
{ "iisSettings": { "windowsAuthentication": false, "anonymousAuthentication": true, "iisExpress": { "applicationUrl": "http://localhost:48555", "sslPort": 0 } }, "profiles": { "WebApplication1": { "commandName": "Project", "dotnetRunMessages": true, "launchBrowser": true, "applicationUrl": "http://localhost:5254", "environmentVariables": { "ASPNETCORE_ENVIRONMENT": "Development" } }, "IIS Express": { "commandName": "IISExpress", "launchBrowser": true, "environmentVariables": { "ASPNETCORE_ENVIRONMENT": "Development" } } }}