2. C-Animation
A Simple Animation in C?
Animation in C in linux follows the steps below
- clear the terminal
- print the frame
- sleep for awhile
- repeat the steps above in a while or for loop
Demonstration
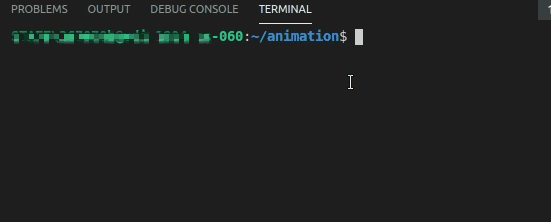
Code
#define _DEFAULT_SOURCE /* Do not remove this. Required for time.h. Must be the on the top */#include <time.h> /* Do not remove this. Required for newSleep */
#include <stdio.h>#include <stdlib.h>#include <string.h>
void newSleep(float sec){ struct timespec ts; ts.tv_sec = (int) sec; ts.tv_nsec = (sec - ((int) sec)) * 1000000000; nanosleep(&ts,NULL);}
void printArray(char* pMap, int iLen){ int i; for (i = 0 ; i < iLen ; i++) { printf("%c", pMap[i]); } printf("\n");}
void progressBarAnimation(char* pMap, int iLen){ int i;
/* Set the object on the array and animate*/ for (i = 0 /* start position */ ; i < iLen ; i++) { system("clear"); /* Clear the terminal */ pMap[i] = '>'; /* Set */ printArray(pMap, iLen); newSleep(1);
}
/* Clear the whole array before exit */ memset(pMap, ' ', sizeof(char) * iLen);}
void movementAnimation(char* pMap, int iLen){ int i;
/* Set the object on the array and animate*/ for (i = 0 /* start position */ ; i < iLen ; i++) { system("clear"); /* Clear the terminal */ pMap[i] = '>'; /* Set */ printArray(pMap, iLen); newSleep(1); pMap[i] = ' '; /* Reset */ }
system("clear"); /* Clear terminal before exit */}
int main(){ int iLen = 10; char* arrcMap = (char*) malloc(sizeof(int*) * iLen); memset(arrcMap, ' ', sizeof(char) * iLen); /* initialize to space char */
progressBarAnimation(arrcMap, iLen); movementAnimation(arrcMap, iLen);
free(arrcMap); return 0;}