1. Command Line Games
1. Make an Object Move Inside a 1d Array
How display an array with a object, and make the object move depending on the keystrokes of the user.
/* Make a object move inside a 1d Array */#include <stdio.h>#include "terminal.h"/* terminal.h is used to enable auto accept chracters from the user (eliminating the need to press Enter key after a chracter input) */
#define FALSE 0#define TRUE 1
void init(char arr[], int len){ int i; for (i = 0 ; i < len ; i++) { arr[i] = '-'; }}
void print(char arr[], int len){ int i;
printf("\n"); for (i = 0 ; i < len ; i++) { printf("%c", arr[i]); }
printf("\n");}
int move_player(char arr[], int len, int prev_player_pos, int player_pos) {
int res = FALSE; if (player_pos < 0 || player_pos >= len) { res = FALSE; } else { arr[prev_player_pos] = '-'; arr[player_pos] = 'X'; res = TRUE; }
return res;}
int main() {
char arr[10]; int len = 10;
/* Place the player object */ int player_pos = 5;
int gameStatus = 2; /* 0: player lost 1: player won 2: neither */ char cUserInput; int success;
init(arr, len);
/* Place the player */ arr[player_pos] = 'X'; print(arr, len);
do { printf("Enter Input (a/d):");
disableBuffer(); scanf(" %c", &cUserInput); enableBuffer();
switch(cUserInput) { /* all movement actions: */ case 'a': /* move to the left */ success = move_player(arr, len, player_pos, player_pos-1); if (success) player_pos = player_pos - 1; break;
case 'd': /* move to the right */ success = move_player(arr, len, player_pos, player_pos+1); if (success) player_pos = player_pos + 1; break;
}
print(arr, len);
} while (gameStatus == 2);
return 0;}
#ifndef TERMINAL_H#define TERMINAL_H
void disableBuffer();void enableBuffer();
#endif
#include<stdio.h>#include<termios.h>#include"terminal.h"
void disableBuffer(){ struct termios mode;
tcgetattr(0, &mode); mode.c_lflag &= ~(ECHO | ICANON); tcsetattr(0, TCSANOW, &mode);}
void enableBuffer(){ struct termios mode;
tcgetattr(0, &mode); mode.c_lflag |= (ECHO | ICANON); tcsetattr(0, TCSANOW, &mode);}
2. Is There Any Sample Code for a Past Assignment?
Yes, there is. Please download the past Assignment Task 01, 2021, Semester 01 (specification) from Blackboard. Read it carefully and refer to the code below:
2021S1 - Task 01
Read the highlights carefully:
- Code comments (short & concise)
- Function header comments (short & concise)
- Modularization of the code (Short functions), 40-60 max lines of code.
- Line width (100 char max)
- Use of #define constants in macros.h (nothing is harded elsewhere)
- Use of function pointers
- Proper indentation of the code
- Low cyclomatic complexity of the code
- No multiple returns, global variables.
- No break, continue statements inside loops (switch statements are allowed).
- No code duplication.
- Header file usage (logical grouping of functions into headers).
- DO NOT include header files inside the header files unless it is absolutely needed.
- Static functions (all methods not listed in headers must be static).
- Meaningful variable names and function names (the name itself indicates what it does without comments).
- Input validation according to the assignment spec.
- 0 warnings, 0 errors during the compilation of the code.
- Valgrind (0 memory leaks, 0 errors) during gameplay & initial stage of validating input.
- Zero crashes (should meet all assignment spec requirements).
- Makefile dependencies are correctly listed (non-dependant headers not included).
- Performance improvement (Refer PERF comment) - Optional
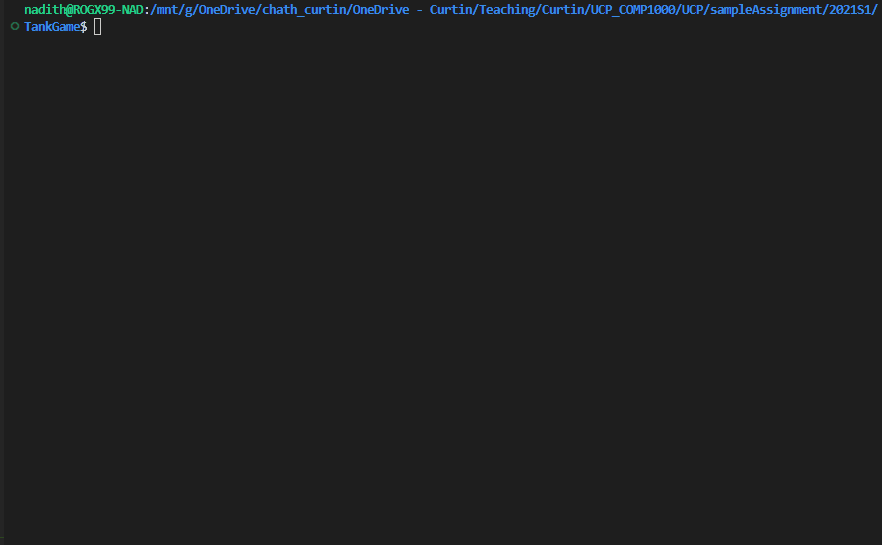
2021S1 - Task 02
Task 02 Highlights:
- All Task 01 Highlights
- Object arrays (enemy, player, bullet) are now converted to structs
- Array indexing for object arrays is eliminated
- All game-related configs are read from a configuration file
- Linked list debug logs to detect all malloced memory has been freed
- File handling errors (file not exists, etc) are handled
- Valgrind (0 memory leaks, 0 errors) during gameplay & initial stage of validating input
- Zero crashes (should meet all assignment spec requirements)
- Makefile dependencies are correctly listed (non-dependant headers not included)