4. FAQ
1. What are variable declarations, definitions, initializations, assignments, function declarations, definitions, invocations? What other terminology is there in C programming?
Please refer to the “Glossary” in Blackboard for the terminology that you need to be aware of in C programming. Getting the right terminology is very important in UCP to understand the advanced concepts of C programming in the later weeks. So make sure you know the terms right!
2. What happens if a variable is declared but not initialized? Any implications?
During variable declaration, the variable will be allocated memory depending on the variable type (In a 64-bit O/S, int - 32 bits, float - 32 bits, double - 64 bits, etc). Since the variable is not initialized, the value it would contain is the value that already exists in the location where the memory is allocated. This is some random garbage value.
3. Do I need to know function recursion?
I’m not from a programming background, but I do have a fair bit of understanding of programming constructs such as if, while, etc. I find it difficult to understand function recursion to perform the lab practical? is it really necessary?
No, it’s not mandatory to know function recursion as long as you know the control structures such as if, while, do-while well in C programming. Function recursion is an alternate way to perform certain tasks easier and faster than writing a while loop.
4. Difference between int main() and void main()
Like any other function, main is also a function but with a special characteristic that the program execution always starts from the ‘main’. ‘int’ and ‘void’ are its return type. So, let’s discuss all of the three one by one. void main – The ANSI standard says “no” to the ‘void main’ and thus using it can be considered wrong. One should stop using the ‘void main’ if doing so. int main – ‘int main’ means that our function needs to return some integer at the end of the execution and we do so by returning 0 at the end of the program. 0 is the standard for the “successful execution of the program”. This is an indication to the underlying O/S which in fact calls the entry point (main() function) in your program. main – In C89, the unspecified return type defaults to int. So, the main is equivalent to int main in C89. But in C99, this is not allowed and thus one must use int main. So, the preferred way is int main.
5. What are %d, %c, etc symbols?
Those are called format specifiers for printf and scanf functions in C. Acts as a place holder and will be replaced with the actual value of the variable when used in printf(…). You will learn more about it in “Arrays and Strings” Lecture.
int num = 10;pritnf("%d", num); /* num is an integer */
Some of the format specifiers are: %d - int (same as %i) %ld - long int (same as %li) %lu - long unsinged int %f - float %lf , %g - double %c - char %s - string %x - hexadecimal
Reference:
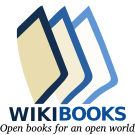
6. What happens if the user inputs a character when an integer (%d) is expected?
Basically, it will not set the character to the integer variable. The variable will be left untouched. Therefore it is important to use the correct placeholder (format specifier) for the right input data type.
int num = 0; /* Initialize the variable to 0 */scanf("%d", &num); /* if user inputs character 'A' */print("%d", num); /* num will still be 0 */
int num; /* num contains some garbage value = 42098 */scanf("%d", &num); /* if user inputs character 'A' */print("%d", num); /* num will be 65 (ASCII value of 'A') */
7. Why do we need to use &variable_name in scanf but not in printf?
Variable has 4 important parts.
- Memory allocation (depends on the variable type)
- Memory address where the memory allocation is
- Name (alias to refer to the allocated memory)
- Value
- & sign will fetch the memory address of a variable given the variable name. Since scanf stores a value from the user in a variable, it needs to know the actual memory address of the variable to locate the location in the memory.
int num;char ch;scanf("%d", &num);scanf(" %c", &ch);/* make sure to keep a space " %c" to consume all the whitespacespreeding the user input character. In contrast, "%d" consumes allwhitespaces by default.Ref: https://eecs.wsu.edu/~cs150/reading/scanf.htm*/
i.e.
Ask for int:buffer: 1\nint is read %dbuffer: \nask for charbuffer: \na\nread char or string, \n is readbuffer: a\n (left in buffer)
Can I pass an integer to a function that expects a char type parameter or vice-versa?
Yes, you can. But remember char has only 8 bits (platform dependant). Therefore you can only store numbers from (-128) to 127 (-2^7 to (2^7) - 1). The signed data types such as (char, int) utilize the 1st bit to indicate the sign of the number. You will learn the representation of signed variables and unsigned variables and the effect of bit-shifting on them in “Misc C” Lecture.
char ch;ch = 'A'; /* All good. 'A' is a char literal */ch = 65; /* All good. 65 is a integer literal */ch = "A"; /* ERROR: "A" is a string literal */
Furthermore, since computers cannot understand characters ‘A’, ‘B’, etc, but numbers (in binary) every character on the keyboard corresponds to a special code called “ASCII code” (or Unicode - the extended version of ASCII). Therefore if you assign a char literal to an integer variable, it means you are assigning the corresponding ASCII value of it. Please refer to the ASCII code table here.
int num = 'A'; /* num will be 65 */char ch = 'A'; /* ch will be 65 */
printf("%d", num); /* prints 65 */printf("%d", ch); /* prints 65 */printf("%c", num); /* prints 'A' */printf("%c", ch); /* prints 'A' */
/*char simply means an allocation of 8 bits (platform dependant)whereasint simply means an allocation of 32 bits (platform dependant)*/
Basically, invoking functions with char type parameters by passing an integer literal or vice versa follow the same concepts explained above.
#include <stdio.h>
void fCh(char ch){ printf("%d", ch); /* prints the numerical value of ch */ printf("%c", ch); /* prints the character corresponds to the value of ch using ASCII conversion table */}
void fInt(int num){ printf("%d", num); /* prints the numerical value of num */ printf("%c", num); /* prints the character corresponds to the value of num using ASCII conversion table */}
int main(){ fCh('A'); fCh(65);
fInt('A'); fInt(65);
return 0;}